How to Use React Context to Simplify State Management
Summary
React Context simplifies state management in large applications by allowing data sharing across components without cumbersome prop drilling. By creating a context with createContext(), setting it with a provider, and accessing it with useContext(), developers can manage state more efficiently. This approach reduces complexity, prevents unnecessary re-renders, and enhances code modularity and readability.
Key insights:
Simplification of Code: React Context removes the need for prop drilling, making the codebase cleaner and easier to manage.
Efficiency in Rendering: It minimizes unnecessary re-renders, optimizing performance by only updating components that consume the affected context.
Enhanced Modularity: Context promotes writing more modular and reusable components by decoupling state management from UI rendering.
Selective Usage: It’s essential to use Context appropriately; overusing it can lead to management issues and overcomplication.
Memoization for Performance: Implementing memoization techniques like
useMemo
anduseCallback
can prevent performance bottlenecks.Error Prevention: Providing default values in Context prevents errors related to undefined context data, ensuring stability.
Testing Strategy: Adequate testing strategies, including the use of libraries like React Testing Library, are crucial for components relying on Context.
Introduction
If you've ever worked on a large React application, you know that managing state can quickly become a complex and challenging task. As the application grows in size and complexity, it becomes increasingly difficult to keep track of all the different pieces of state and how they interact with one another.
Fortunately, React provides a solution to this problem in the form of React Context. In this article, we'll explore what React Context is, how it works, and how you can use it to simplify state management in your web app projects.
Understanding React Context
React Context is a feature that allows you to share data between components without having to pass props manually at every level. It provides a way to pass data down the component tree without having to use props, which can be especially useful for passing state data.
To use React Context, you first need to create a context object using the createContext() method. This context object will be used to hold the state data that you want to share between components. For example:

Once you have a context object, you can use it to set up a provider component that will make the context available to child components. The provider component is responsible for setting the initial state of the context and passing it down to child components:
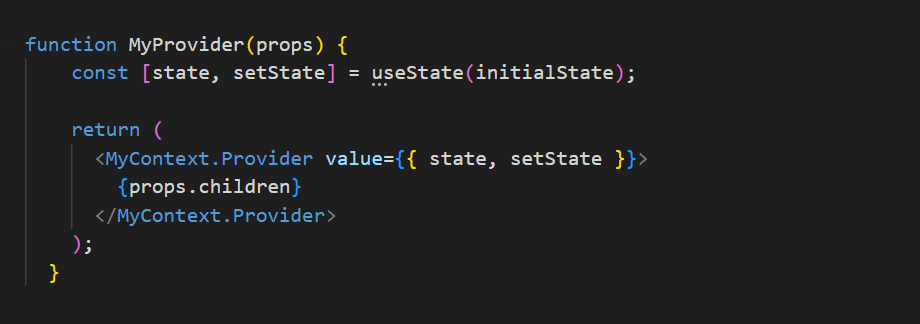
In this example, we're using the useState() hook to set up an initial state for the context. We then wrap our child components in the provider component and pass the state and setState functions down through the context.
Implementing React Context
Now that we've set up our context and provider component, we can start using it to manage state in our application. To access the state data in child components, we can use the useContext() hook:
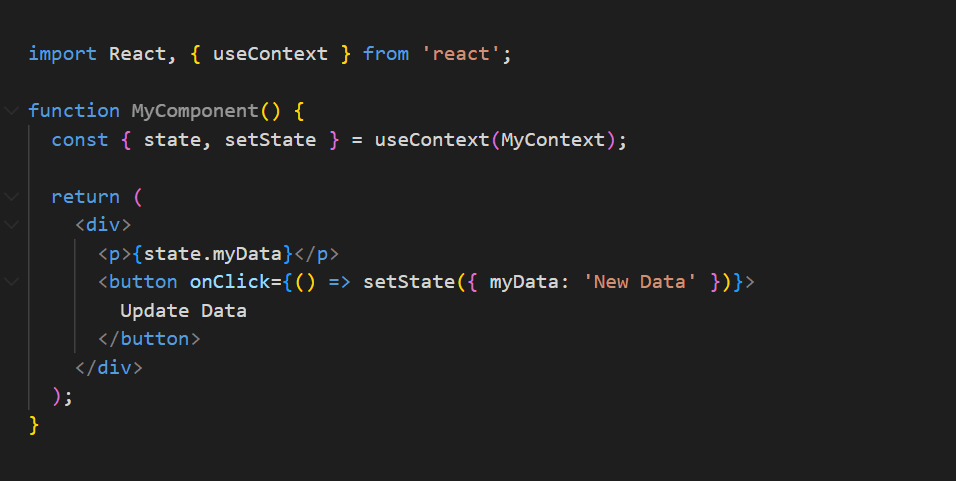
In this example, we're using the useContext() hook to access the state and setState functions that we defined in our provider component. We can then use these functions to read and update the state data.
Benefits of Using React Context
Using React Context for state management has a number of advantages. One of the biggest benefits is that it simplifies your code and reduces complexity. By using a centralized context object to manage state, you don't have to worry about passing props down through multiple levels of components. This can make your code much easier to read and maintain.
Another advantage of using React Context for state management is that it can improve performance by reducing the number of renders. When state changes in a component, React will automatically re-render that component and all of its child components. By using a centralized context object, you can reduce the number of renders that occur in your application.
Finally, using React Context for state management can help you write more modular and reusable code. By separating state management from component rendering, you can create more focused and specialized components that are easier to reuse throughout your application.
Common Mistakes to Avoid
While using React Context for state management can be a powerful tool, there are a few common pitfalls to avoid including:
Overusing Context - It can be tempting to use Context for every piece of state in your application, but this can quickly become unwieldy and make your code more difficult to manage. Be selective in what you choose to manage with Context and consider other state management options, like Redux, for more complex scenarios.
Not Using Memoization - Since Context can cause all components that consume it to re-render, it's important to use memoization techniques to prevent unnecessary re-renders. Use the useMemo() or useCallback() hooks to memoize expensive computations or functions that depend on the Context data.
Not Providing Default Values - If a component tries to consume a Context that has no value provided, it will throw an error. To prevent this, provide default values for your Contexts that can be used if no other value is provided.
Testing Complexity - Testing components that rely on Context can be complex, as it may be difficult to mock or manipulate the Context data in your tests. Consider using a testing library like React Testing Library or Enzyme to simplify your testing approach.
To Summarize
In this article, we've explored how to use React Context to simplify state management in large applications. By creating a context object, setting up a provider component, and using the useContext() hook, you can pass state data down the component tree without having to use props manually.
Using React Context can simplify your code, reduce complexity, and improve performance in your application. However, be careful not to overuse Context and avoid common pitfalls like not using memoization, not providing default values, and testing complexity.
With these best practices in mind, you'll be well on your way to using React Context to manage state in your own large applications.