How to Use TypeScript with React
May 11, 2023
Guest User
Summary
TypeScript can significantly improve your React development workflow by catching potential bugs early and providing robust type safety. This article guides you through setting up a React project with TypeScript, covering the basics of TypeScript syntax, and demonstrating how to write components, manage state, and define props using TypeScript.
Key insights:
TypeScript enhances React development by catching potential bugs early and improving workflow.
Setting up a React project with TypeScript using Create React App is straightforward.
TypeScript’s static typing helps in writing self-documenting code.
It improves state management with libraries like Redux or MobX.
TypeScript provides better editor support and type safety compared to React's built-in PropTypes.
Introduction
If you want to improve your React development workflow and ensure that potential bugs are caught before they become a problem, TypeScript is an excellent tool to consider. In this article, we'll walk you through the process of setting up a React project with TypeScript and demonstrate how to write components, manage state, and define props using this powerful language.
With TypeScript, you can unlock a whole new level of productivity and confidence in your React development. Whether you're an experienced developer looking to streamline your workflow or a newcomer looking to learn best practices, it can provide a solid foundation for building scalable, maintainable React applications.
Setting up Your Project
The first step in using TypeScript with React is to create a new React project with TypeScript using Create React App. This will give you a basic project structure that you can build upon.
To create a new project, run the following command in your terminal:

This will create a new React project in the my-app
directory and set it up to use TypeScript.
Once your project is created, you'll need to install some additional dependencies to enable TypeScript support. Run the following command in your terminal:

This will install TypeScript and the necessary type definitions for Node.js, React, React DOM, and Jest.
TypeScript Basics
Before we dive into using TypeScript with React, let's take a brief look at some of the basics of TypeScript syntax and type annotations. TypeScript is a statically-typed superset of JavaScript that adds optional type annotations to the language. These annotations can help catch potential bugs before runtime and make your code more self-documenting.
Here's an example of a simple TypeScript function that takes two numbers as arguments and returns their sum:

In this example, we define a functional component called Greeting
that takes a single prop called name
. The Props
interface defines the shape of the props that the component expects, and the React.FC<Props>
type annotation indicates that this component is a function component that expects props of type Props
.
State Management with TypeScript
In addition to writing components with TypeScript, you can also use TypeScript to manage state in your React application. For example, if you're using a state management library like Redux or MobX, you can define the types for your state and actions to ensure type safety and prevent errors.
Here's an example of defining a type for the state in a Redux store:

In this example, we define an interface called AppState
that describes the shape of our state object. This can then be used to create a typed version of the Redux store, like this:
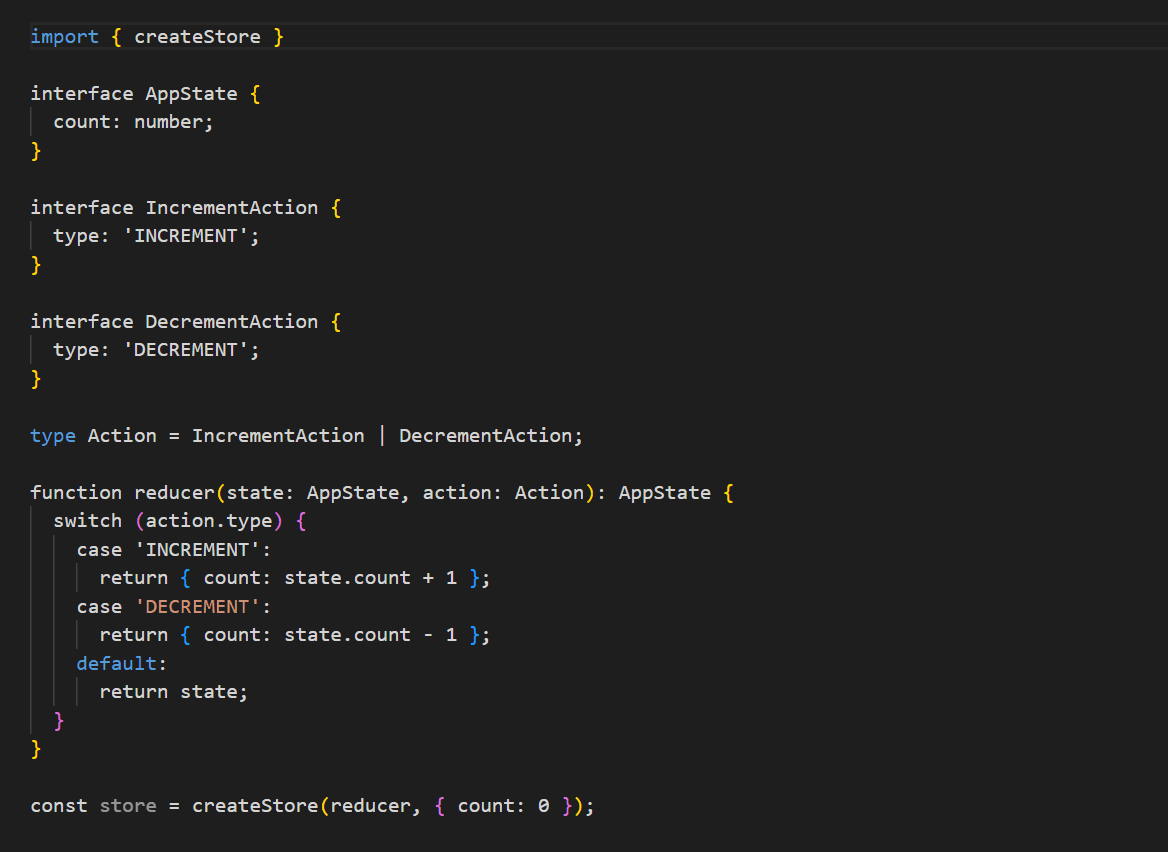
In this example, we define two action interfaces (IncrementAction
and DecrementAction
) that describe the shape of the actions that our Redux store can dispatch. We then define a union type called Action
that includes both of these action types.
Finally, we define a reducer function that takes the current state and an action as arguments, and returns the new state based on the action type. The createStore
function from the redux
library is used to create a typed version of the Redux store.
Props and PropTypes
Another area where TypeScript can improve your React development workflow is in defining and using props. While React has a built-in PropTypes
system for validating props, using TypeScript can provide additional benefits like improved code editor support and type safety.
The code snippet below is an example of defining props for a React component in TypeScript:
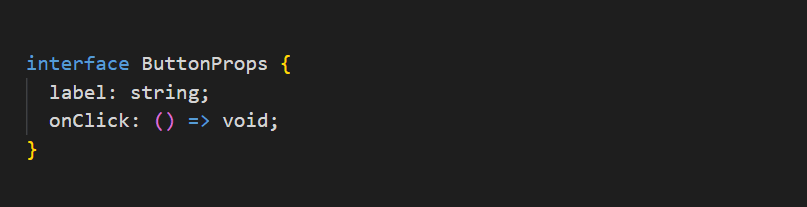
In this example, we define an interface called ButtonProps
that describes the shape of the props that the component expects. This includes a label
prop of type string
and an onClick
prop of type () => void
.
In a Nutshell
Using TypeScript with React can provide many benefits for your development workflow, including improved code quality, catch potential bugs before runtime and better editor support. In this article, we walked through the process of setting up a React project with TypeScript and demonstrated how to write components, manage state, and define props using TypeScript. By using TypeScript with React, you can take your development skills to the next level and write more robust, maintainable code.